Running into an imresize error can be frustrating. You’re working on resizing an image in MATLAB or Python, and boom! The error pops up. Let’s break down how to fix this issue in a simple way.
Why Does the Imresize Error Happen?
There are a few reasons this error might show up:
- The function doesn’t exist in your version of MATLAB.
- The package containing imresize isn’t installed in Python.
- You passed the wrong types of inputs.
Don’t worry! We’ll go through each problem and fix them step by step.
Fixing the Imresize Error in MATLAB
If MATLAB throws an error when you try to use imresize, here’s what to do:
1. Check If You Have the Image Processing Toolbox
The imresize function is part of the Image Processing Toolbox. If you don’t have it, MATLAB won’t recognize the function.
ver
Run this command in MATLAB. If you don’t see “Image Processing Toolbox” in the list, you need to install it.
2. Reinstall the Toolbox
- Go to MATLAB’s Add-Ons manager.
- Search for “Image Processing Toolbox”.
- Click Install.
Once installed, restart MATLAB and try running your code again.
3. Check Your Function Syntax
Make sure you’re passing the correct arguments. The correct format is:
outputImage = imresize(inputImage, scale);
Or, if resizing to a specific size:
outputImage = imresize(inputImage, [newHeight, newWidth]);
Fixing the Imresize Error in Python
If you’re using Python and see an imresize error, it’s likely because imresize was removed from SciPy.
1. Install the Required Library
In older versions of SciPy, you could use:
from scipy.misc import imresize
But in newer versions, imresize is gone. Instead, use OpenCV or PIL:
pip install opencv-python
2. Use OpenCV Instead
Replace your imresize code with:
import cv2
import numpy as np
image = cv2.imread('image.jpg')
resized_image = cv2.resize(image, (new_width, new_height))
Now your code should work!
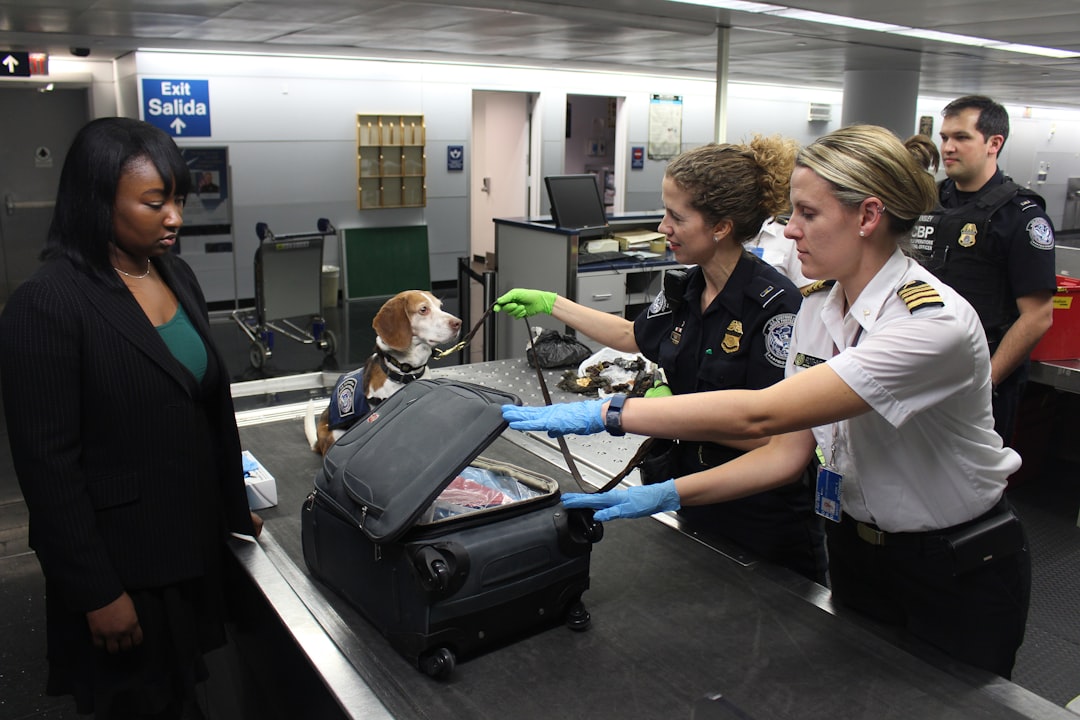
3. Or Use PIL (Pillow)
If you prefer PIL, try this:
from PIL import Image
image = Image.open('image.jpg')
resized_image = image.resize((new_width, new_height))
Additional Tips
- Double-check your image’s format before resizing.
- If using NumPy arrays, ensure they contain valid pixel values (0-255).
- Restart your environment after installing new libraries.
Final Thoughts
The imresize error is common, but easy to fix. Whether you’re in MATLAB or Python, you now have the tools to resolve the issue. Just check your toolbox, install the right packages, and use alternative functions if needed.
Happy coding!
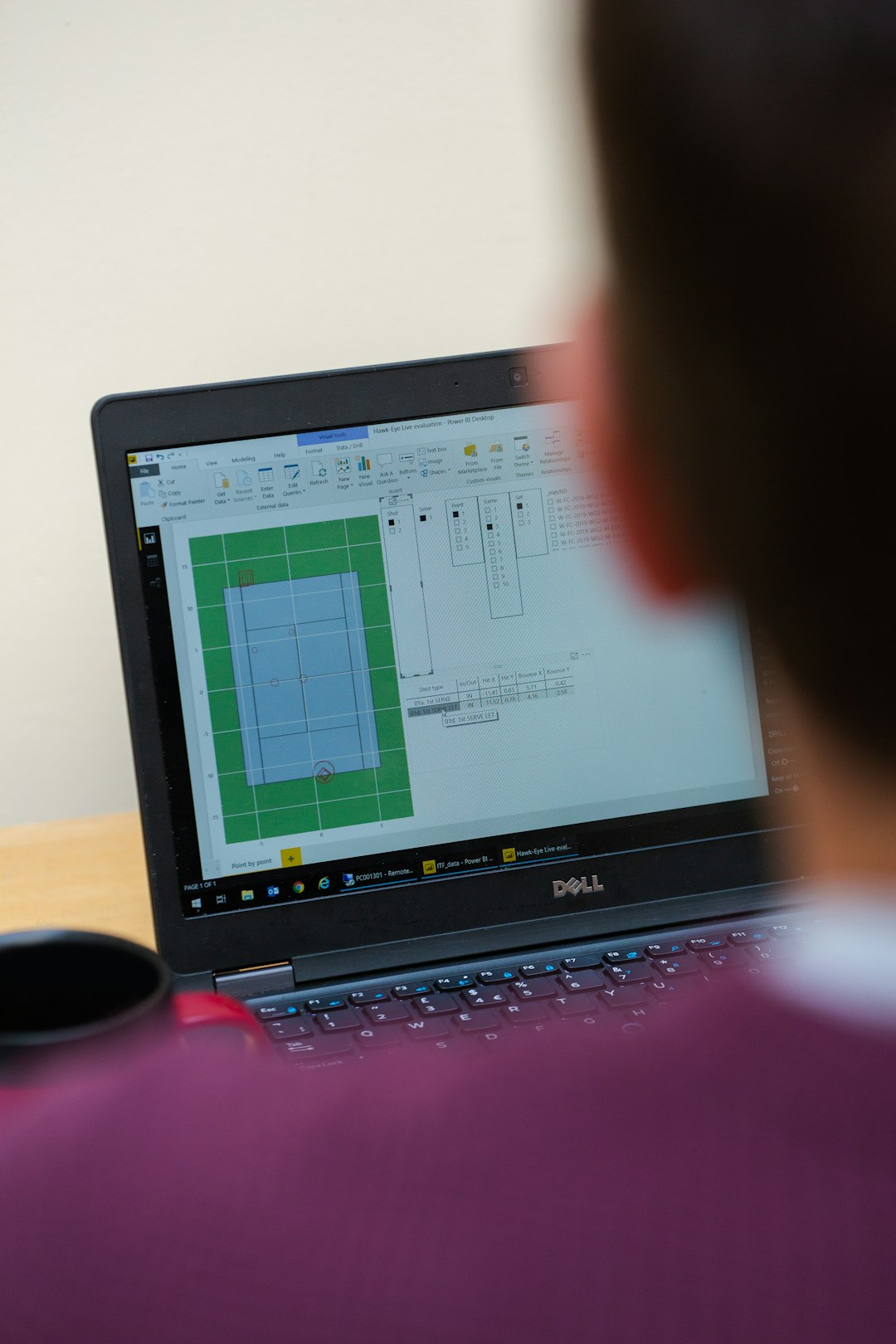